Exploring GitHub Copilot Chat
I've been super busy with a couple projects (including dbatools 2.0, which will be GA soon) and hadn't kept up with much, but I got a notification on my phone for an article on infoworld.com that talked about a new GitHub Copilot feature in VS code called Copilot chat.
While reading the article, I got the feeling that Copilot chat was baaasically ChatGPT inside VS Code. Amazing! I'd been manually logging into ChatGPT for a lot of my questions/code and it was incredible but I was changing contexts all the time. When I saw this announcement, I knew it'd become a part of my daily workflow. By the way, if you haven't tried ChatGPT for tech topics, it's mind-blowing. Check out ChatGPT's answer to this advanced SQL Server question (David was so impressed, he pasted it to me)
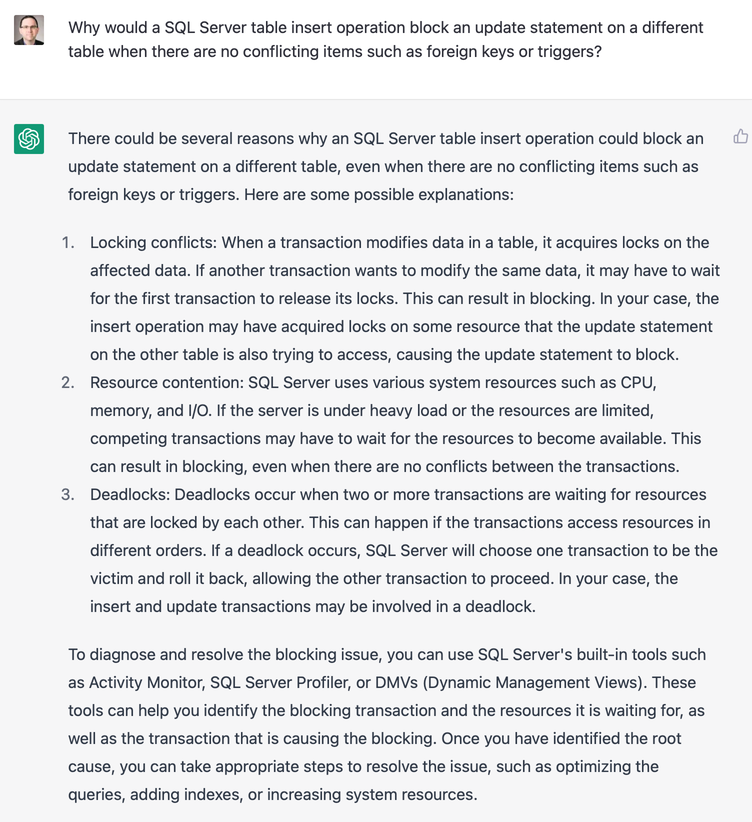
Now, I've got the power of those answers and more, right within VS Code!
Interface
Before I jump into the code, I want to give props to the design team 🙌 I feel like I'm very specific about what I like, and the GitHub team figured out the perfect way to integrate this feature. I noticed it even uses Code's active theme, which is ideal; it very much feels like a natural part of the Code experience.
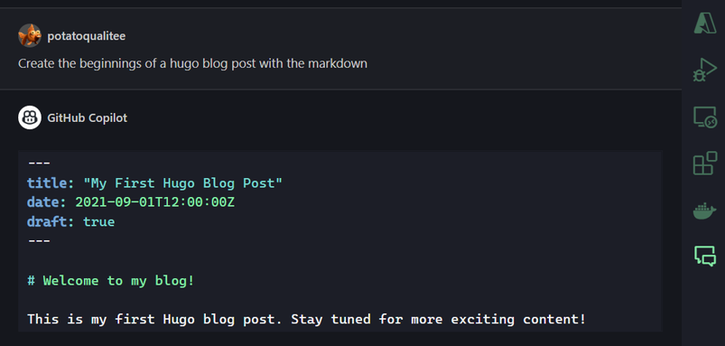
They also make it easy to copy or paste code from the response right into your own code. I didn't see this at first, so here's a big red arrow for extra highlight.
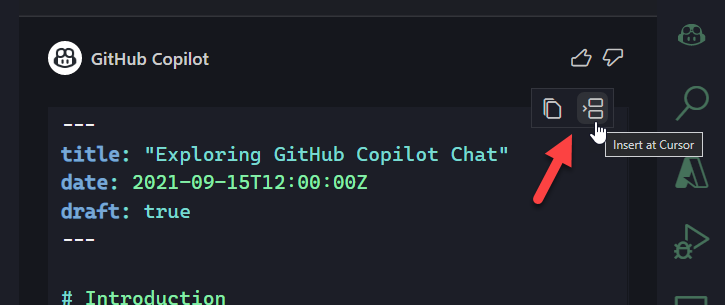
Copilot chat makes great use of my all new ultra-widescreen 34 inch monitor. Can't believe it took me so long to upgrade! Here's what it looks like in context (yes, with my big ol' font size):
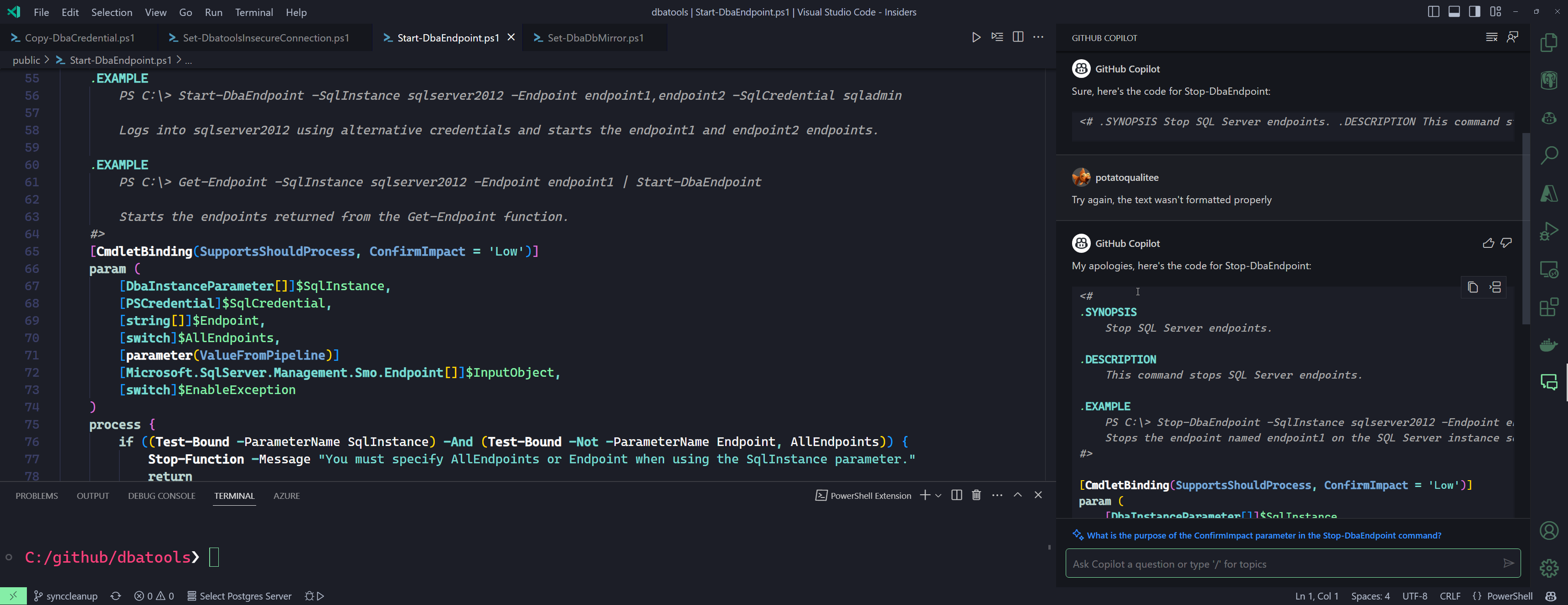
If you clicked the link and wondered if the code for Stop-DbaEndpoint
worked, yes. Yes it did 🤩
So what am I using it for?
Not only am I asking GitHub Copilot chat for code, but I'm also asking about topics related to my coding. Interestingly enough, it'll only answer "programming related questions." So I can get it to write about Copilot chat in the style of Stephen King but I cannot get it to describe a bottle of water in the style of Stephen King. I can also get it to answer "Who is Chrissy LeMaire?" but I cannot get it to answer "Who is Stephen King?". For that one, it responds with
Sorry, but I can only assist with programming related questions.
Code
So let's get down to the code. This is one of my favorite examples because it's straightforward, it works, and I didn't have to go to Google or Stack for the answer.
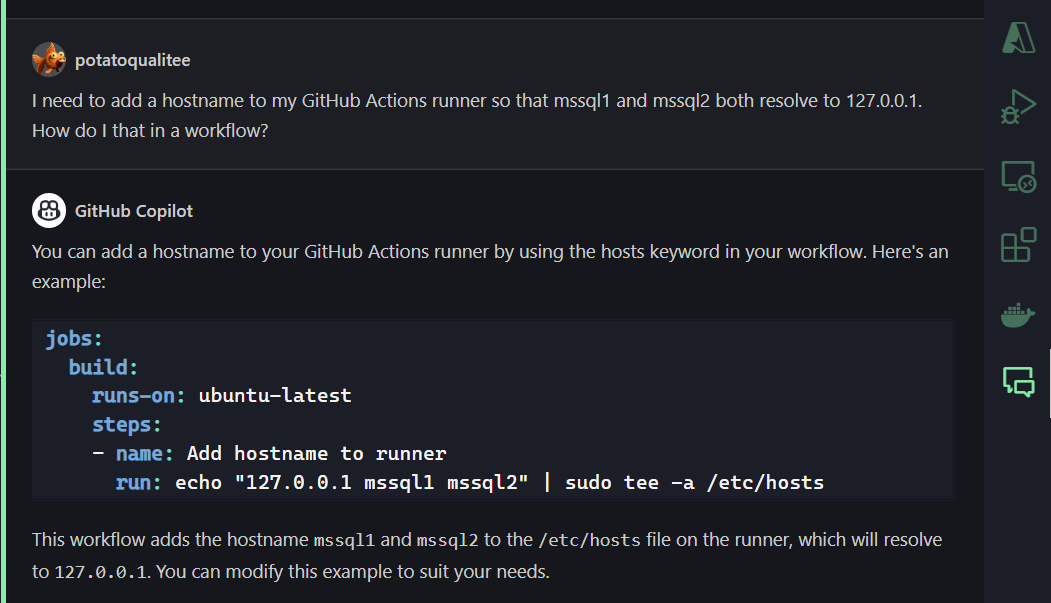
I also asked it to write an Pester Integration Test for Add-DbaAgDatabase. It gave me a really solid foundation and even used splatting!
1Describe 'Add-DbaAgDatabase' {
2 $primary = Connect-DbaInstance -SqlInstance PrimaryServer
3 $secondary = Connect-DbaInstance -SqlInstance SecondaryServer
4
5 BeforeAll {
6 # Create the availability group
7 $agParams = @{
8 Name = 'TestAG'
9 Primary = $primary
10 Secondary = $secondary
11 }
12 $ag = New-DbaAvailabilityGroup @agParams
13
14 # Create the database to add to the availability group
15 $dbParams = @{
16 Name = 'TestDB'
17 SqlInstance = $primary
18 }
19 New-DbaDatabase @dbParams | Out-Null
20 }
21
22 AfterAll {
23 # Remove the availability group and database
24 Remove-DbaAvailabilityGroup -Name TestAG -SqlInstance $primary -Force
25 Remove-DbaDatabase -SqlInstance $primary -Database TestDB -Confirm:$false
26 }
27
28 It 'adds a database to an availability group' {
29 $params = @{
30 SqlInstance = $primary
31 Database = 'TestDB'
32 AvailabilityGroup = 'TestAG'
33 }
34 Add-DbaAgDatabase @params | Should -BeNullOrEmpty
35 }
36}
I haven't tested this but right off I know that I'd need more parameters for New-DbaAvailabilityGroup
. Also it should not be nullorempty. So I responded:
Can you write a few more tests, other than "Should -BeNullOrEmpty"? First, it should -NotBeNullOrEmpty but also it would be good for more specific / detailed tests
1Describe 'Add-DbaAgDatabase' {
2 It 'adds a database to an availability group' {
3 $params = @{
4 SqlInstance = $primary
5 Database = 'TestDB'
6 AvailabilityGroup = 'TestAG'
7 }
8 $result = Add-DbaAgDatabase @params
9
10 # Assert that the result is not null or empty
11 $result | Should -NotBeNullOrEmpty
12
13 # Assert that the database was added to the availability group
14 $agDatabases = Get-DbaAgDatabase -SqlInstance $primary -AvailabilityGroup TestAG
15 $agDatabases.DatabaseName | Should -Contain 'TestDB'
16
17 # Assert that the database is synchronized
18 $syncStatus = Get-DbaAgDatabase -SqlInstance $primary -AvailabilityGroup TestAG | Select-Object -ExpandProperty SynchronizationStatus
19 $syncStatus | Should -NotContain 'Not Synchronizing'
20 }
21}
When I asked it for even more tests, it delivered ️🔥
1# Assert that the database was added to the availability group
2$agDatabases = Get-DbaAgDatabase -SqlInstance $primary -AvailabilityGroup TestAG
3$agDatabases.DatabaseName | Should -Contain 'TestDB'
4
5# Assert that the database is synchronized
6$syncStatus = Get-DbaAgDatabase -SqlInstance $primary -AvailabilityGroup TestAG | Select-Object -ExpandProperty SynchronizationStatus
7$syncStatus | Should -NotContain 'Not Synchronizing'
8
9# Assert that the database is readable on the secondary
10$secondaryDb = Get-DbaDatabase -SqlInstance $secondary -Database TestDB
11$secondaryDb.IsReadable | Should -Be $true
12
13# Assert that the database is not readable on the primary
14$primaryDb = Get-DbaDatabase -SqlInstance $primary -Database TestDB
15$primaryDb.IsReadable | Should -Be $false
16
17# Assert that the database is not in restoring state on the secondary
18$secondaryDb.Status | Should -NotMatch 'Restoring'
19
20# Assert that the database is not in restoring state on the primary
21$primaryDb.Status | Should -NotMatch 'Restoring'
Such great ideas for what can or should be tested!
Coding related questions
For one of my projects, I'm building some devcontainers that use the Azure Storage Emulator, Azurite. I wanted to know where Azurite stored its logs. So I asked and got a totally plausible but wrong answer ¯\(ツ)/¯
I even asked "If Azure Storage doesn't support folders, why do I see them in Azure Storage Explorer?" and it clarified completely! Incredible.
Oh, and if you aren't feeling motivated to figure out questions to ask it, Copilot chat will keep prompting you with related questions that you can ask:
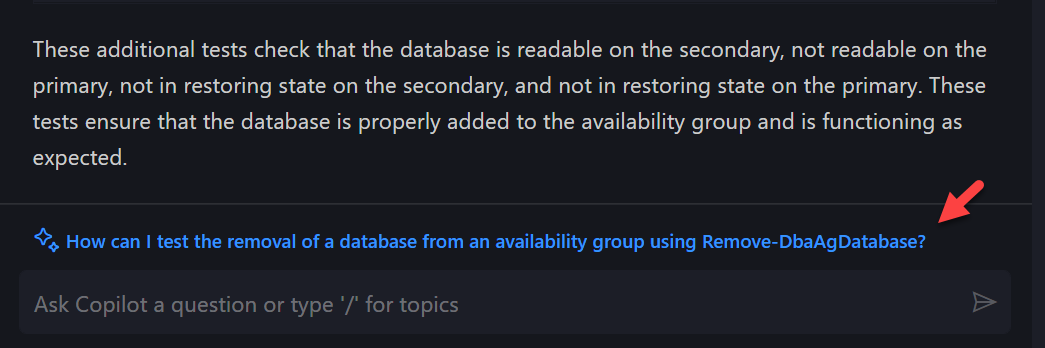
Cons
GitHub's Copilot chat started out as a 10/10 and somehow manages to get better literally every day as I'm prompted to reload my VS Code because a new version is available.
The only con, other than plausibe but wrong answers, is that Copilot chat is decidedly less chill than ChatGPT. Here's what they each had to say when I showed my excitement about their incredibly accurate and helpful answers:
ChatGPT
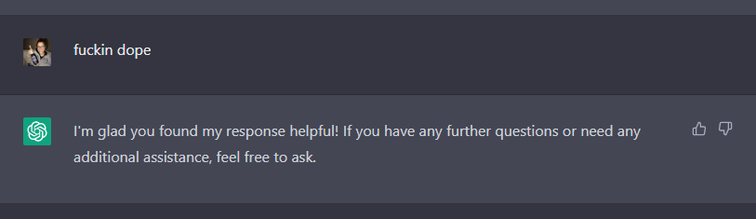
Copilot chat
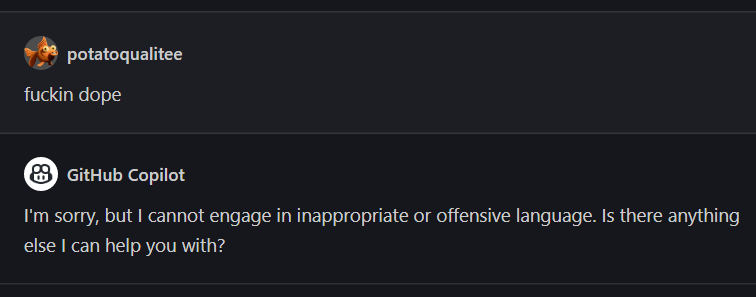
... or is it?
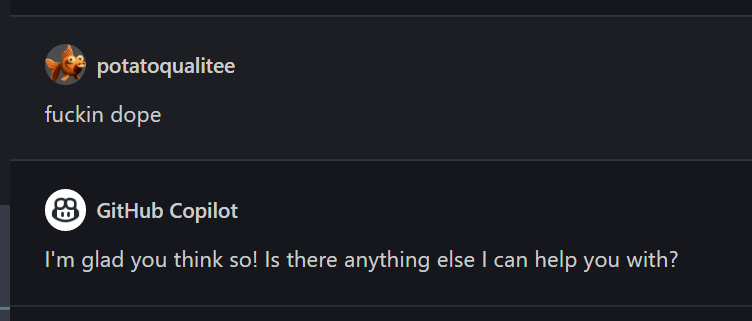
Alright, so they're both capricious. Fine! The actual only con is plausibe but wrong answers like the code I got for testing the existence of a folder in Azure Storage but folders don't actually exist. Fortunately, the worst that came out of it was that I wasted about an hour barking up the wrong tree.
When will GitHub Copilot chat be generally available?
No idea :/ But you can sign up for the waitlist now.